
Category Archives: java
Guide to ThreadLocalRandom in Java
Udemy - Programming Languages
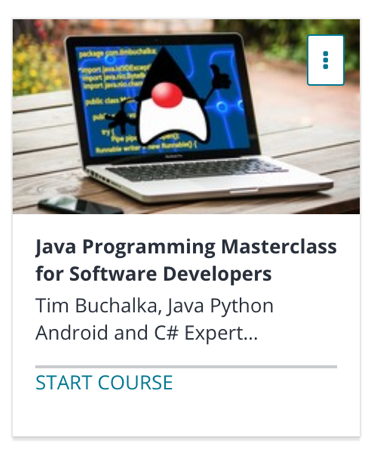
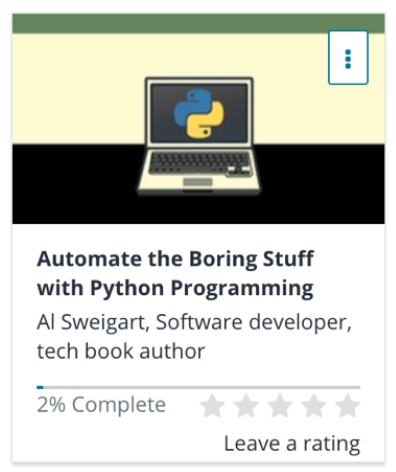
CUvids COS 126 Videos (Paid)
InformIT COS 226 Videos
InformIT COS 126 Videos
COS 226 Data Structures Programs github
https://github.com/kevin-wayne/algs4/tree/master/src/main/java/edu/princeton/cs/algs4
COS 126 Java Programming
https://introcs.cs.princeton.edu/java/code/
Java Programs in the Textbook
Standard libraries.
Here are the standard input and output libraries that we use throughout the textbook.
Programs in the textbook.
Below is a table of the Java programs in the textbook. Click on the program name to access the Java code; click on the reference number for a brief description; read the textbook for a full discussion. You can download all of the programs as introcs.jar and the data as introcs-data.zip; you can also download all of the programs and data as the IntelliJ project IntroCS.zip.
1 | ELEMENTS OF PROGRAMMING | |
---|---|---|
1.1.1 | HelloWorld.java | Hello, World |
1.1.2 | UseArgument.java | using a command-line argument |
1.2.1 | Ruler.java | string concatenation example |
1.2.2 | IntOps.java | integer multiplication and division |
1.2.3 | Quadratic.java | quadratic formula |
1.2.4 | LeapYear.java | leap year |
1.2.5 | RandomInt.java | casting to get a random integer |
1.3.1 | Flip.java | flippling a fair coin |
1.3.2 | TenHellos.java | your first while loop |
1.3.3 | PowersOfTwo.java | computing powers of 2 |
1.3.4 | DivisorPattern.java | your first nested loops |
1.3.5 | HarmonicNumber.java | harmonic numbers |
1.3.6 | Sqrt.java | Newton's method |
1.3.7 | Binary.java | converting to binary |
1.3.8 | Gambler.java | gambler's ruin simulation |
1.3.9 | Factors.java | factoring integers |
1.4.1 | Sample.java | sampling without replacement |
1.4.2 | CouponCollector.java | coupon collector simulation |
1.4.3 | PrimeSieve.java | sieve of Eratosthenes |
1.4.4 | SelfAvoidingWalk.java | self-avoiding random walks |
1.5.1 | RandomSeq.java | generating a random sequence |
1.5.2 | TwentyQuestions.java | interactive user input |
1.5.3 | Average.java | averaging a stream of numbers |
1.5.4 | RangeFilter.java | a simple filter |
1.5.5 | PlotFilter.java | standard input-to-drawing filter |
1.5.6 | BouncingBall.java | bouncing ball |
1.5.7 | PlayThatTune.java | digital signal processing |
1.6.1 | Transition.java | computing the transition matrix |
1.6.2 | RandomSurfer.java | simulating a random surfer |
1.6.3 | Markov.java | mixing a Markov chain |
2 | FUNCTIONS | |
2.1.1 | Harmonic.java | harmonic numbers (revisited) |
2.1.2 | Gaussian.java | Gaussian functions |
2.1.3 | Coupon.java | coupon collector (revisited) |
2.1.4 | PlayThatTuneDeluxe.java | play that tune (revisited) |
2.2.1 | StdRandom.java | random number library |
2.2.2 | StdArrayIO.java | array I/O library |
2.2.3 | IFS.java | iterated function systems |
2.2.4 | StdStats.java | data analysis library |
2.2.5 | StdStats.java | data analysis library |
2.2.6 | Bernoulli.java | Bernoulli trials |
2.3.1 | Euclid.java | Euclid's algorithm |
2.3.2 | TowersOfHanoi.java | towers of Hanoi |
2.3.3 | Beckett.java | Gray code |
2.3.4 | Htree.java | recursive graphics |
2.3.5 | Brownian.java | Brownian bridge |
2.3.6 | LongestCommonSubsequence.java | longest common subsequence |
2.4.1 | Percolation.java | percolation scaffolding |
2.4.2 | VerticalPercolation.java | vertical percolation |
2.4.3 | PercolationVisualizer.java | percolation visualization client |
2.4.4 | PercolationProbability.java | percolation probability estimate |
2.4.5 | Percolation.java | percolation detection |
2.4.6 | PercolationPlot.java | adaptive plot client |
3 | OBJECT ORIENTED PROGRAMMING | |
3.1.1 | PotentialGene.java | identifying a potential gene |
3.1.2 | AlbersSquares.java | Albers squares |
3.1.3 | Luminance.java | luminance library |
3.1.4 | Grayscale.java | converting color to grayscale |
3.1.5 | Scale.java | image scaling |
3.1.6 | Fade.java | fade effect |
3.1.7 | Cat.java | concatenating files |
3.1.8 | StockQuote.java | screen scraping for stock quotes |
3.1.9 | Split.java | splitting a file |
3.2.1 | Charge.java | charged-particle data type |
3.2.2 | Stopwatch.java | stopwatch data type |
3.2.3 | Histogram.java | histogram data type |
3.2.4 | Turtle.java | turtle graphics data type |
3.2.5 | Spiral.java | spira mirabilis |
3.2.6 | Complex.java | complex number data type |
3.2.7 | Mandelbrot.java | Mandelbrot set |
3.2.8 | StockAccount.java | stock account data type |
3.3.1 | Complex.java | complex number data type (revisited) |
3.3.2 | Counter.java | counter data type |
3.3.3 | Vector.java | spatial vector data type |
3.3.4 | Sketch.java | document sketch data type |
3.3.5 | CompareDocuments.java | similarity detection |
3.4.1 | Body.java | gravitational body data type |
3.4.2 | Universe.java | n-body simulation |
4 | DATA STRUCTURES | |
4.1.1 | ThreeSum.java | 3-sum problem |
4.1.2 | DoublingTest.java | validating a doubling hypothesis |
4.2.1 | Questions.java | binary search (20 questions) |
4.2.2 | Gaussian.java | bisection search |
4.2.3 | BinarySearch.java | binary search (in a sorted array) |
4.2.4 | Insertion.java | insertion sort |
4.2.5 | InsertionTest.java | doubling test for insertion sort |
4.2.6 | Merge.java | mergesort |
4.2.7 | FrequencyCount.java | frequency counts |
4.3.1 | ArrayStackOfStrings.java | stack of strings (array) |
4.3.2 | LinkedStackOfStrings.java | stack of strings (linked list) |
4.3.3 | ResizingArrayStackOfStrings.java | stack of strings (resizing array) |
4.3.4 | Stack.java | generic stack |
4.3.5 | Evaluate.java | expression evaluation |
4.3.6 | Queue.java | generic queue |
4.3.7 | MM1Queue.java | M/M/1 queue simulation |
4.3.8 | LoadBalance.java | load balancing simulation |
4.4.1 | Lookup.java | dictionary lookup |
4.4.2 | Index.java | indexing |
4.4.3 | HashST.java | hash table |
4.4.4 | BST.java | binary search tree |
4.4.5 | DeDup.java | dedup filter |
– | ST.java | symbol table data type |
– | SET.java | set data type |
4.5.1 | Graph.java | graph data type |
4.5.2 | IndexGraph.java | using a graph to invert an index |
4.5.3 | PathFinder.java | shortest-paths client |
4.5.4 | PathFinder.java | shortest-paths implementation |
4.5.5 | SmallWorld.java | small-world test |
4.5.6 | Performer.java | performer–performer graph |
Exercise solutions.
Here is a list of solutions to selected coding exercises.
1 | ELEMENTS OF PROGRAMMING | |
---|---|---|
1.1.1 | TenHelloWorlds.java | ten Hello, Worlds |
1.1.5 | UseThree.java | three command-line arguments |
1.2.20 | SumOfTwoDice.java | sum of two dice |
1.2.23 | SpringSeason.java | is month and day in Spring? |
1.2.25 | WindChill.java | compute wind chill factor |
1.2.26 | CartesianToPolar.java | Cartesian to polar coordinates |
1.2.29 | DayOfWeek.java | compute day of week from date |
1.2.30 | Stats5.java | average, min, max of 5 random numbers |
1.2.34 | ThreeSort.java | sort three integers |
1.2.35 | Dragon.java | dragon curve of order 5 |
1.3.8 | FivePerLine.java | print integers five per line |
1.3.11 | FunctionGrowth.java | table of functions |
1.3.12 | DigitReverser.java | reverse digits |
1.3.13 | Fibonacci.java | Fibonacci numbers |
1.3.15 | SeriesSum.java | convergent sum |
1.3.31 | Ramanujan.java | taxicab numbers |
1.3.32 | ISBN.java | ISBN checksum |
1.3.38 | Sin.java | sine function via Taylor series |
1.3.41 | MonteHall.java | Monte Hall problem |
1.4.2 | HugeArray.java | creating a huge array |
1.4.10 | Deal.java | deal poker hands |
1.4.13 | Transpose.java | tranpose a square matrix |
1.4.25 | InversePermutation.java | compute inverse permutation |
1.4.26 | Hadamard.java | compute Hadamard matrix |
1.4.30 | Minesweeper.java | create Minesweeper board |
1.4.33 | RandomWalkers.java | N random walkers |
1.4.35 | Birthdays.java | birthday problem |
1.4.37 | BinomialCoefficients.java | binomial coefficients |
1.5.1 | MaxMin.java | max and min from standard input |
1.5.3 | Stats.java | mean and stddev from standard input |
1.5.5 | LongestRun.java | longest consecutive run from stdin |
1.5.11 | WordCount.java | word count from standard input |
1.5.15 | Closest.java | closest point |
1.5.18 | Checkerboard.java | draw a checkerboard |
1.5.21 | Rose.java | draw a rose |
1.5.22 | Banner.java | animate a text banner |
1.5.31 | Spirograph.java | draw spirograph |
1.5.32 | Clock.java | animate a clock |
1.5.33 | Oscilloscope.java | simulate an oscilloscope |
2 | FUNCTIONS | |
2.1.4 | ArrayEquals.java | are two integer arrays equal? |
2.1.30 | BlackScholes.java | Black-Scholes option valuation |
2.1.32 | Horner.java | Horner's method to evaluate a polynomial |
2.1.33 | Benford.java | Benford's law |
2.1.38 | Calendar.java | create a calendar |
2.2.1 | Gaussian.java | overloaded gaussian distribution functions |
2.2.2 | Hyperbolic.java | hyperbolic trig functions |
2.2.4 | StdRandom.java | shuffle an array of doubles |
2.2.6 | StdArrayIO.java | array IO methods |
2.2.11 | Matrix.java | matrix operations |
2.2.12 | MarkovSquaring.java | page rank via matrix squaring |
2.2.14 | StdRandom.java | exponential random variable |
2.3.14 | AnimatedHtree.java | animated H-tree |
2.3.15 | IntegerToBinary.java | integer to binary conversion |
2.3.17 | Permutations.java | all permutations |
2.3.18 | PermutationsK.java | all permutations of size k |
2.3.19 | Combinations.java | all combinations |
2.3.20 | CombinationsK.java | all combinations of size k |
2.3.22 | RecursiveSquares.java | recursive squares |
2.3.24 | GrayCode.java | Gray code |
2.3.26 | AnimatedHanoi.java | animated Towers of Hanoi |
2.3.29 | Collatz.java | Collatz function |
2.3.30 | BrownianIsland.java | Brownian island |
2.3.31 | PlasmaCloud.java | plama cloud |
2.3.32 | McCarthy.java | McCarthy's 91 function |
2.3.33 | Tree.java | fractal tree |
2.4.15 | PercolationDirectedNonrecursive.java | directed percolation |
Copyright © 2000–2019 Robert Sedgewick and Kevin Wayne. All rights reserved.
An Idiots Guide to Really Really Bad Programming
https://www.codeproject.com/kb/scrapbook/bad_programming.aspx
Bottom of page links:
Introduction
I have noticed that certain individuals persist in writing good clear code that is well documented and easy to understand. So I thought it was about time to draw the main techniques of really really bad programming together into a single reference document. Remember the most important two things when writing truly awful software are to include as many subtle bugs as possible and to make the code really confusing so that it is hard to track those devious little bugs down. That way you can annoy both users and programmers to the maximum extent.
If you follow these simple rules, I promise you that you will never be far from users’ and other programmers’ thoughts.
1. Turn Off All Compiler Warnings
If you want to write really awful code, this is a great place to start. After all, you do not want that pesky compiler nagging you all day. All real programmers turn the warnings off completely.
2. Variables
Beginners should ensure that all variables are global. It is much easier to keep them all together in one place. As you become more advanced, add local variables with the same names as the globals, this will allow you to create subtle bugs with ease.
3. Functions
Avoid using functions wherever possible. It is much easier to write one single enormous long passage of code than going through the tiresome process of trying to divide it into separate functions and having the tedious job of passing parameters to them.
4. Cut and Paste is your Friend
Avoid using loops at all costs, it might take longer to cut and paste a section of code over and over, but it is well worth it when you consider the hours of fun for anyone checking each and every repeat of that code for subtle variations.
5. Variable Names for Beginners
You can have so much fun with variable names, try using the most meaningless name that you can think of, Fred is one of my personal favourites. But always include a smattering of semi meaningful names to keep other programmers guessing.
6. Advanced Variable Name Techniques
Ideally try to think of names that are very similar for as many entirely different variables as you can. Another great idea is to create two variables with the same name, but one ending in ‘1’ and the other in ‘l’, these are very easy to confuse at a glance and should get other programmers really guessing. Example: Slopel and Slope1. However, you can achieve the best results by occasionally using a label to mean the exact opposite of what people would assume, a classic example is to use the label Horizontal to mean Vertical and vice versa, that will really please any reader of your code, causing hours of amusement and pleasure as they try to unravel it.
7. Initialising
Play programmers bingo by allowing all of your variables to start with whatever value the memory had in from the last application. Great fun!
8. Bounds Checking
Allow the user to enter whatever values they want, if they enter a stupid value the program may crash. So? What do they expect?
9. Comments
It is best for beginners to avoid comments at all times, they waste valuable space and take ages to type. As you become more advanced, you can begin by adding comments which are entirely useless, because they state the obvious such as:
i++; // increment i
However, the most advanced comments will be as cryptic as possible, such as:
i++; //check inside the chicken string
10. Layout
It takes real dedication to make your software layout truly bad. The most important thing is NEVER EVER be consistent. Whatever layout philosophy you choose, be sure to change it regularly. Advanced programmers should remember to use the same layout for long enough for any other programmer to become accustomed to it before changing to something entirely different.
11. Hungarian Notation
This is entirely optional, but for best results use a smattering of Hungarian Notation, thus annoying everyone (those who like it AND those who don't) and of course include a few incorrect uses just to make things more interesting.
12. Lastly Never Ever Test
Don't bother to test your code, just wait for people to complain, that way you will find out which are the most commonly occurring bugs first! Brilliant!
I have tried to cover most aspects of Really Really Bad Programming, but I am sure many of you can think of a few more. And don't be down hearted if after a while you start to slip into good habits, it doesn't take much effort to get back to those bad bad ways.
Disclaimer
I am not in any way suggesting that I never write bad code, nor am I suggesting that anyone else at CodeProject does. Just having a little fun!
License
This article, along with any associated source code and files, is licensed under The Code Project Open License (CPOL)
40 awesome programming quotes
Interface and Inheritance - Default Methods
Inmutable Objects
Collected Java Practices
Java File: Reading and Writing Files in Java
Online Programming Visualizer
Lejos EV3 Tutorials
Java Programming Tutorial - thenewboston
Java and python assessments
Java Fill Polygon
Beginning Graphics (using BreezyGUI)
The New Boston
java - system.out.printf
UML Editor
Java printf cheat sheet
zetcode
ZetCode brings tutorials for programmers in various areas. The main are Graphical User Interfaces, databases, and programming languages. The website's mission is to provide competent, quick and easy to understand tutorials for modern-day technologies.
News
PostgreSQL Ruby tutorial written (August 2, 2015)
PostgreSQL C tutorial written (July 30, 2015)
GTK+ 2 tutorial cleaned and updated (July 20, 2015)
JavaFX tutorial written (June 26, 2015)
Java SWT tutorial cleaned and updated (June 5, 2015)
Advanced Java Swing e-book published (May 23, 2015)
Windows API cleaned and updated (March 26, 2015)
PyQt5 tutorial written (January 28, 2015)
Tcl tutorial cleaned and updated (January 25, 2015)
SQLite tutorial updated (November 18, 2014)
SQLite C tutorial written (November 12, 2014)
Coming
Arduino, JavaScript tutorial, Mono tutorial, PyGame tutorial, CSS tutorial, C tutorial, C++ tutorial, Firebird tutorial, PostgreSQL tutorial, JQuery tutorial, Python 3 tutorial, D tutorial, Apache Tomcat tutorial, Gambas tutorial, Java Games e-book, Advanced Qt e-book, Flex tutorial, Java Servlets tutorial, Java IO tutorial, LINQ tutorial, Ant tutorial, SQLAlchemy tutorial, AWK tutorial, Bash tutorial
Java 2D games tutorial
java fx
Java Image 2D
Related examples in the same category
1. Image size Image size
2. Image demo Image demo
3. Getting the Color Model of an Image
4. Filtering the RGB Values in an Image
5. Create a filter that can modify any of the RGB pixel values in an image.
6. This filter removes all but the red values in an image
7. Load and draw image
8. Paint an Icon Paint an Icon
9. Image Processing: Brightness and Contrast Image Processing: Brightness and Contrast
10. Image with mouse drag and move event Image with mouse drag and move event
11. Image Animation and Thread Image Animation and Thread
12. Image Color Gray Effect Image Color Gray Effect
13. Image Buffering Image Buffering
14. Image Effect: Combine Image Effect: Combine
15. AffineTransform demo AffineTransform demo
16. Image Effect: Rotate Image using DataBuffer Image Effect: Rotate Image using DataBuffer
17. Image Effect: Sharpen, blur Image Effect: Sharpen, blur
18. Image scale
19. Image crop
20. Demonstrating the Drawing of an Image with a Convolve Operation
21. Demonstrating Use of the Image I/O Library
22. Adding Image-Dragging Behavior
23. Sending Image Objects through the Clipboard Sending Image Objects through the Clipboard
24. Anti Alias Anti Alias
25. Image Operations Image Operations
26. Image Viewer Image Viewer
27. Get the dimensions of the image; these will be non-negative
28. Standalone Image Viewer - works with any AWT-supported format
29. Toolkit.getImage() which works the same in either Applet or Application
30. Double Buffered Image
31. Graband Fade: displays image and fades to black
32. Graband Fade with Rasters
33. Rotate Image 45 Degrees
34. Convert java.awt.image.BufferedImage to java.awt.Image
35. Filter image by multiplier its red, green and blue color
36. Drags within the image Drags within the image
37. TYPE_INT_RGB and TYPE_INT_ARGB are typically used
38. Pixels from a buffered image can be modified
39. Calculation of the mean value of an image with Raster
40. Use PixelGrabber class to acquire pixel data from an Image object
41. Rendered Image
42. Image Panel
43. Image Utils
44. Returns an image resource.
45. Create Gradient Image
46. Create Gradient Mask
47. Create Translucent Image
48. Make Raster Writable
49. A frame that displays an image
50. Optimized version of copyData designed to work on Integer packed data with a SinglePixelPackedSampleModel
51. Various image processing operations. Various image processing operations.
52. This program demonstrates the transfer of images between a Java application and the system clipboard. This program demonstrates the transfer of images between a Java application and the system clipboard.
53. Scales down an image into a box of maxSideLenght x maxSideLength.
54. Adding watermark to an image
55. Scale Image
56. Crop Image
57. Fit Image
58. Converts a java.awt.Image into an array of pixels
59. Creates a scaled copy of the source image.
60. Provides useful methods for converting images from one colour depth to another.
61. Reads an image in a file and creates a thumbnail in another file.
62. Make image Transparency Make image Transparency
63. Clips the input image to the specified shape
64. Image Sorter frame
65. Returns an ImageIcon, or null if the path was invalid.
66. Create new image from source image
67. check if image supported
68. Get supported image format
69. get image thumbnail
70. get image orientation type
71. get fixing preview image
Java Transformations in 2D
Javafx tutorial
StdIn.java notes
public static void main(String[] args) {
final double G = 6.67 * Math.pow(10,-11);
final double T = Double.parseDouble(args[0]);
final double DELTAT = Double.parseDouble(args[1]);
String tablePlanet = "mercury";
int numPlanets = Integer.parseInt(StdIn.readString());
double universeRadius = Double.parseDouble(StdIn.readString());
How to install LWJGL
Java file update
The Coding Universe
TRAER.PHYSICS 3.0
N-body Simulations - Code
N-body Simulations - Code Part 1
Object-oriented programming
In general, programming languages have built in objects, such as integers, float point numbers, booleans, arrays, etc. that can be used very easily by the user. These allow programmers to code virtually whatever they need, but it is often easier to create objects that better suit the application that is being coded. The following examples are written in Java, so a small background would be useful. Even though the syntax may be slightly different, other programming languages such as C++ and Python can accomplish identical tasks.
It would be nice to declare a body, b, with the following parameters:
Body b = new Body(double rx, double ry, double vx, double vy, double mass, Color color)
This is accomplished, in Java, using the code below. Comments are prefaced by //.
import java.awt.Color; public class Body { private static final double G = 6.673e-11; // gravitational constant private static final double solarmass=1.98892e30; public double rx, ry; // holds the cartesian positions public double vx, vy; // velocity components public double fx, fy; // force components public double mass; // mass public Color color; // color (for fun) // create and initialize a new Body public Body(double rx, double ry, double vx, double vy, double mass, Color color) { this.rx = rx; this.ry = ry; this.vx = vx; this.vy = vy; this.mass = mass; this.color = color; } // update the velocity and position using a timestep dt public void update(double dt) { vx += dt * fx / mass; vy += dt * fy / mass; rx += dt * vx; ry += dt * vy; } // returns the distance between two bodies public double distanceTo(Body b) { double dx = rx - b.rx; double dy = ry - b.ry; return Math.sqrt(dx*dx + dy*dy); } // set the force to 0 for the next iteration public void resetForce() { fx = 0.0; fy = 0.0; } // compute the net force acting between the body a and b, and // add to the net force acting on a public void addForce(Body b) { Body a = this; double EPS = 3E4; // softening parameter (just to avoid infinities) double dx = b.rx - a.rx; double dy = b.ry - a.ry; double dist = Math.sqrt(dx*dx + dy*dy); double F = (G * a.mass * b.mass) / (dist*dist + EPS*EPS); a.fx += F * dx / dist; a.fy += F * dy / dist; } // convert to string representation formatted nicely public String toString() { return "" + rx + ", "+ ry+ ", "+ vx+ ", "+ vy+ ", "+ mass; } }
This defines the Body object that we will use to store information about bodies in our simulation. This file would be saved in a file Body.java and compiled into a class Body.class. The class file should reside in the same directory as all other class files.
On the next page we will create the main class that uses these methods to run the simulation.
N-body Simulations- Code Part 2
Using our Body object
Recall that by creating a new class, we have a Body object that can be called using:
Body b = new Body(double rx, double ry, double vx, double vy, double mass, Color color)
Now, we want to use the brute-force method to update the positions and velocities of the bodies. Here's the code for an applet that we'll see at the end of this tutorial.
BruteForce.java
// tell the compiler where to find the methods you will use. // required when you create an applet import java.applet.*; // required to paint on screen import java.awt.*; import java.awt.event.*; //Start the applet and define a few necessary variables public class BruteForce extends Applet { public int N = 100; public Body bodies[]= new Body[10000]; public TextField t1; public Label l1; public Button b1; public Button b2; public boolean shouldrun=false; // The first time we call the applet, this function will start public void init() { startthebodies(N); t1=new TextField("100",5); b2=new Button("Restart"); b1=new Button("Stop"); l1=new Label("Number of bodies:"); ButtonListener myButtonListener = new ButtonListener(); b1.addActionListener(myButtonListener); b2.addActionListener(myButtonListener); add(l1); add(t1); add(b2); add(b1); } // This method gets called when the applet is terminated. It stops the code public void stop() { shouldrun=false; } //Called by the applet initally. It can be executed again by calling repaint(); public void paint(Graphics g) { g.translate(250,250); //Originally the origin is in the top right. Put it in its normal place //check if we stopped the applet, and if not, draw the particles where they are if (shouldrun) { for(int i=0; i<N; i++) { g.setColor(bodies[i].color); g.fillOval((int) Math.round(bodies[i].rx*250/1e18),(int) Math.round(bodies[i].ry*250/1e18),8,8); } //go through the Brute Force algorithm (see the function below) addforces(N); //go through the same process again until applet is stopped repaint(); } } //the bodies are initialized in circular orbits around the central mass. //This is just some physics to do that public static double circlev(double rx, double ry) { double solarmass=1.98892e30; double r2=Math.sqrt(rx*rx+ry*ry); double numerator=(6.67e-11)*1e6*solarmass; return Math.sqrt(numerator/r2); } //Initialize N bodies with random positions and circular velocities public void startthebodies(int N) { double radius = 1e18; // radius of universe double solarmass=1.98892e30; for (int i = 0; i < N; i++) { double px = 1e18*exp(-1.8)*(.5-Math.random()); double py = 1e18*exp(-1.8)*(.5-Math.random()); double magv = circlev(px,py); double absangle = Math.atan(Math.abs(py/px)); double thetav= Math.PI/2-absangle; double phiv = Math.random()*Math.PI; double vx = -1*Math.signum(py)*Math.cos(thetav)*magv; double vy = Math.signum(px)*Math.sin(thetav)*magv; //Orient a random 2D circular orbit if (Math.random() <=.5) { vx=-vx; vy=-vy; } double mass = Math.random()*solarmass*10+1e20; //Color the masses in green gradients by mass int red = (int) Math.floor(mass*254/(solarmass*10+1e20)); int blue = (int) Math.floor(mass*254/(solarmass*10+1e20)); int green = 255; Color color = new Color(red, green, blue); bodies[i] = new Body(px, py, vx, vy, mass, color); } //Put the central mass in bodies[0]= new Body(0,0,0,0,1e6*solarmass,Color.red);//put a heavy body in the center } //Use the method in Body to reset the forces, then add all the new forces public void addforces(int N) { for (int i = 0; i < N; i++) { bodies[i].resetForce(); //Notice-2 loops-->N^2 complexity for (int j = 0; j < N; j++) { if (i != j) bodies[i].addForce(bodies[j]); } } //Then, loop again and update the bodies using timestep dt for (int i = 0; i < N; i++) { bodies[i].update(1e11); } } public static double exp(double lambda) { return -Math.log(1 - Math.random()) / lambda; } public boolean action(Event e,Object o) { N = Integer.parseInt(t1.getText()); if (N>1000) { t1.setText("1000"); N=1000; } startthebodies(N); repaint(); return true; } public class ButtonListener implements ActionListener{ public void actionPerformed(ActionEvent evt) { // Get label of the button clicked in event passed in String arg = evt.getActionCommand(); if (arg.equals("Restart")) { shouldrun=true; N = Integer.parseInt(t1.getText()); if (N>1000) { t1.setText("1000"); N=1000; } startthebodies(N); repaint(); } else if (arg.equals("Stop")) stop(); } } }
And that's it! It's pretty simple once we have the Body object defined. This is easily embedded in a webpage using the
<applet code>
You can skip to the Applets page to see this one in action, or head to the Next section of programming, where we will look at the Barnes-Hut algorithm.
N-body Simulations- Code Part 3
Implementing the Barnes-Hut Algorithm: A Quadrant Class
In the Introduction we talked about an object called a Barnes-Hut tree. This is one of two additional parts to the Barnes-Hut algorithm. The other is the concept of a quadrant (in 2D) or an octant (in 3D). For coding simplicity, we will create a quadrant-based Barnes-Hut tree. It is not difficult to add additional nodes if need be.
To create a quadrant object, the code goes as follows (again, comments preceded by //):
//A quadrant object that can self-subdivide. Important for creating a Barnes-Hut tree, since it holds quadrants. public class Quad { private double xmid, ymid, length; //Create a square quadrant with a given length and midpoints (xmid,ymid) public Quad(double xmid, double ymid, double length) { this.xmid=xmid; this.ymid=ymid; this.length=length; } //How long is this quadrant? public double length() { return length; } //Check if the current quadrant contains a point public boolean contains(double xmid, double ymid) { if (xmid<=this.xmid+this.length/2.0 && xmid>=this.xmid-this.length/2.0 && ymid<=this.ymid+this.length/2.0 && ymid>=this.ymid-this.length/2.0) { return true; } else { return false; } } //Create subdivisions of the current quadrant // Subdivision: Northwest quadrant public Quad NW() { Quad newquad = new Quad(this.xmid-this.length/4.0, this.ymid+this.length/4.0,this.length/2.0); return newquad; } // Subdivision: Northeast quadrant public Quad NE() { Quad newquad = new Quad(this.xmid+this.length/4.0, this.ymid+this.length/4.0,this.length/2.0); return newquad; } // Subdivision: Southwest quadrant public Quad SW() { Quad newquad = new Quad(this.xmid-this.length/4.0, this.ymid-this.length/4.0,this.length/2.0); return newquad; } // Subdivision: Southeast quadrant public Quad SE() { Quad newquad = new Quad(this.xmid+this.length/4.0, this.ymid-this.length/4.0,this.length/2.0); return newquad; } } One more thing that is useful to update now is the Body class so we can check if the body is in a certain quadrant. To do this, all we have to do is add the following method, which takes advantage of the methods we just defined in the Quad class. public boolean in(Quad q) { return q.contains(this.rx,this.ry); } }
Now we have a data structure to hold Bodies, but what we really need is the Barnes-Hut tree that hold quadrants and contain information about the bodies they contain. The next section will do just that.
N-body Simulations- Code Part 4
Implementing the Barnes-Hut Algorithm: A Barnes-Hut Tree
Now, we need to create a Barnes-Hut tree that will hold the quadrants and bodies, and allow us to approximate far-away bodies by their total and center of mass.
The code is fairly complex and its methods are non-trivial, so it is necessary to really look through the code to see what is happening. The complexity draws for the recursive methods that are mentioned in the comments.
To create a Barnes-Hut tree, the code goes as follows (again, comments preceded by //):
import java.awt.Color; public class BHTree { private Body body; // body or aggregate body stored in this node private Quad quad; // square region that the tree represents private BHTree NW; // tree representing northwest quadrant private BHTree NE; // tree representing northeast quadrant private BHTree SW; // tree representing southwest quadrant private BHTree SE; // tree representing southeast quadrant //Create and initialize a new bhtree. Initially, all nodes are null and will be filled by recursion //Each BHTree represents a quadrant and a body that represents all bodies inside the quadrant public BHTree(Quad q) { this.quad=q; this.body=null; this.NW=null; this.NE=null; this.SW=null; this.SE=null; } //If all nodes of the BHTree are null, then the quadrant represents a single body and it is "external" public Boolean isExternal(BHTree t) { if (t.NW==null && t.NE==null && t.SW==null && t.SE==null) return true; else return false; } //We have to populate the tree with bodies. We start at the current tree and recursively travel through the branches public void insert(Body b) { //If there's not a body there already, put the body there. if (this.body==null) { this.body=b; } //If there's already a body there, but it's not an external node //combine the two bodies and figure out which quadrant of the //tree it should be located in. Then recursively update the nodes below it. else if (this.isExternal(this)==false) { this.body=b.add(this.body,b); Quad northwest = this.quad.NW(); if (b.in(northwest)) { if (this.NW==null) {this.NW= new BHTree(northwest);} NW.insert(b); } else { Quad northeast = this.quad.NE(); if (b.in(northeast)) { if (this.NE==null) {this.NE= new BHTree(northeast);} NE.insert(b); } else { Quad southeast = this.quad.SE(); if (b.in(southeast)) { if (this.SE==null) {this.SE= new BHTree(southeast);} SE.insert(b); } else { Quad southwest = this.quad.SW(); if(this.SW==null) {this.SW= new BHTree(southwest);} SW.insert(b); } } } } //If the node is external and contains another body, create BHTrees //where the bodies should go, update the node, and end //(do not do anything recursively) else if (this.isExternal(this)) { Body c = this.body; Quad northwest = this.quad.NW(); if (c.in(northwest)) { if (this.NW==null) {this.NW= new BHTree(northwest);} NW.insert(c); } else { Quad northeast = this.quad.NE(); if (c.in(northeast)) { if (this.NE==null) {this.NE= new BHTree(northeast);} NE.insert(c); } else { Quad southeast = this.quad.SE(); if (c.in(southeast)) { if (this.SE==null) {this.SE= new BHTree(southeast);} SE.insert(c); } else { Quad southwest = this.quad.SW(); if(this.SW==null) {this.SW= new BHTree(southwest);} SW.insert(c); } } } this.insert(b); } } //Start at the main node of the tree. Then, recursively go each branch //Until either we reach an external node or we reach a node that is sufficiently //far away that the external nodes would not matter much. public void updateForce(Body b) { if (this.isExternal(this)) { if (this.body!=b) b.addForce(this.body); } else if (this.quad.length()/(this.body.distanceTo(b))<2){ b.addForce(this.body); } else { if (this.NW!=null) this.NW.updateForce(b); if (this.SW!=null) this.SW.updateForce(b); if (this.SE!=null) this.SE.updateForce(b); if (this.NE!=null) this.NE.updateForce(b); } } // convert to string representation for output public String toString() { if(NE != null || NW!=null || SW!=null || SE!=null) return "*" + body + "\n" + NW + NE + SW + SE; else return " " + body + "\n"; } }
Now these are all the data types we need to run the algorithm. It's obvious that they are much less trivial than the Body data type, but they are essential if we want to cut down run time. Next, we put it all together in our main class that actually runs the simulation.
N-body Simulations- Code Part 5
Implementing the Barnes-Hut Algorithm: Main Method
Now that we have all our objects described, it is fairly straight-forward to run the simulation. Below is the commented code--the function that has been changed is addforces(int N), which has been updated to use the Barnes-Hut method. Other than that difference, our object-oriented approach has allowed this code to look almost identical to the Brute Force case. One big difference is that now, instead of invoking a loop to add all the forces on a body, we now just call the updateForce(Body b) method from our BHTree class, which takes, on average, Log(N) time to run, versus a loop that would take N.
// tell the compiler where to find the methods you will use. // required when you create an applet import java.applet.*; // required to paint on screen import java.awt.*; import java.awt.event.*; //Start the applet and define a few necessary variables public class BarnesHut extends Applet { public int N = 100; public Body bodies[]= new Body[10000]; public TextField t1; public Label l1; public Button b1; public Button b2; public boolean shouldrun=false; Quad q = new Quad(0,0,2*1e18); // The first time we call the applet, this function will start public void init() { startthebodies(N); t1=new TextField("100",5); b2=new Button("Restart"); b1=new Button("Stop"); l1=new Label("Number of bodies:"); ButtonListener myButtonListener = new ButtonListener(); b1.addActionListener(myButtonListener); b2.addActionListener(myButtonListener); add(l1); add(t1); add(b2); add(b1); } // This method gets called when the applet is terminated. It stops the code public void stop() { shouldrun=false; } //Called by the applet initally. It can be executed again by calling repaint(); public void paint(Graphics g) { g.translate(250,250); //Originally the origin is in the top right. Put it in its normal place //check if we stopped the applet, and if not, draw the particles where they are if (shouldrun) { for(int i=0; i<N; i++) { g.setColor(bodies[i].color); g.fillOval((int) Math.round(bodies[i].rx*250/1e18),(int) Math.round(bodies[i].ry*250/1e18),8,8); } //go through the Barnes-Hut algorithm (see the function below) addforces(N); //repeat repaint(); } } //the bodies are initialized in circular orbits around the central mass. //This is just some physics to do that public static double circlev(double rx, double ry) { double solarmass=1.98892e30; double r2=Math.sqrt(rx*rx+ry*ry); double numerator=(6.67e-11)*1e6*solarmass; return Math.sqrt(numerator/r2); } //Initialize N bodies public void startthebodies(int N) { double radius = 1e18; // radius of universe double solarmass=1.98892e30; for (int i = 0; i < N; i++) { double px = 1e18*exp(-1.8)*(.5-Math.random()); double py = 1e18*exp(-1.8)*(.5-Math.random()); double magv = circlev(px,py); double absangle = Math.atan(Math.abs(py/px)); double thetav= Math.PI/2-absangle; double phiv = Math.random()*Math.PI; double vx = -1*Math.signum(py)*Math.cos(thetav)*magv; double vy = Math.signum(px)*Math.sin(thetav)*magv; //Orient a random 2D circular orbit if (Math.random() <=.5) { vx=-vx; vy=-vy; } double mass = Math.random()*solarmass*10+1e20; //Color a shade of blue based on mass int red = (int) Math.floor(mass*254/(solarmass*10+1e20)); int blue = 255; int green = (int) Math.floor(mass*254/(solarmass*10+1e20)); Color color = new Color(red, green, blue); bodies[i] = new Body(px, py, vx, vy, mass, color); } bodies[0]= new Body(0,0,0,0,1e6*solarmass,Color.red);//put a heavy body in the center } //The BH algorithm: calculate the forces public void addforces(int N) { BHTree thetree = new BHTree(q); // If the body is still on the screen, add it to the tree for (int i = 0; i < N; i++) { if (bodies[i].in(q)) thetree.insert(bodies[i]); } //Now, use out methods in BHTree to update the forces, //traveling recursively through the tree for (int i = 0; i < N; i++) { bodies[i].resetForce(); if (bodies[i].in(q)) { thetree.updateForce(bodies[i]); //Calculate the new positions on a time step dt (1e11 here) bodies[i].update(1e11); } } } //A function to return an exponential distribution for position public static double exp(double lambda) { return -Math.log(1 - Math.random()) / lambda; } public boolean action(Event e,Object o) { N = Integer.parseInt(t1.getText()); if (N>10000) { t1.setText("10000"); N=10000; } startthebodies(N); repaint(); return true; } public class ButtonListener implements ActionListener{ public void actionPerformed(ActionEvent evt) { // Get label of the button clicked in event passed in String arg = evt.getActionCommand(); if (arg.equals("Restart")) { shouldrun=true; N = Integer.parseInt(t1.getText()); if (N>10000) { t1.setText("10000"); N=10000; } startthebodies(N); repaint(); } else if (arg.equals("Stop")) stop(); } } }
And that's it! The objects were more complicated, but the end code is quite simple and elegant. To see both codes in action, go to the Applets page. Here, you can play around with N, the number of bodies, to watch the dynamics and get a sense for the how computationally intensive each method is.
Site Map
N-body Simulations
A friendly place for programming greenhorns
Conway's game of life lacklan
Brandom Horn
175 MC questions to prepare for the APCS Exam
Binary Trees in Java - New Think Tank
Java Notes - Binary Trees
Objects First with Java Video Notes
Object First with Java BlueJ
Program Style Guide
Lightweight java game library
PU Java Algorithms and Clients
Linear congruential generator
C
#include/* always assuming int is at least 32 bits */ int rand(); int rseed = 0; inline void srand(int x) { rseed = x; } #ifndef MS_RAND #define RAND_MAX ((1U << 31) - 1) inline int rand() { return rseed = (rseed * 1103515245 + 12345) & RAND_MAX; } #else /* MS rand */ #define RAND_MAX_32 ((1U << 31) - 1) #define RAND_MAX ((1U << 15) - 1) inline int rand() { return (rseed = (rseed * 214013 + 2531011) & RAND_MAX_32) >> 16; } #endif/* MS_RAND */ int main() { int i; printf("rand max is %d\n", RAND_MAX); for (i = 0; i < 100; i++) printf("%d\n", rand()); return 0; }
C++
#include//-------------------------------------------------------------------------------------------------- using namespace std; //-------------------------------------------------------------------------------------------------- class mRND { public: void seed( unsigned int s ) { _seed = s; } protected: mRND() : _seed( 0 ), _a( 0 ), _c( 0 ), _m( 2147483648 ) {} int rnd() { return( _seed = ( _a * _seed + _c ) % _m ); } int _a, _c; unsigned int _m, _seed; }; //-------------------------------------------------------------------------------------------------- class MS_RND : public mRND { public: MS_RND() { _a = 214013; _c = 2531011; } int rnd() { return mRND::rnd() >> 16; } }; //-------------------------------------------------------------------------------------------------- class BSD_RND : public mRND { public: BSD_RND() { _a = 1103515245; _c = 12345; } int rnd() { return mRND::rnd(); } }; //-------------------------------------------------------------------------------------------------- int main( int argc, char* argv[] ) { BSD_RND bsd_rnd; MS_RND ms_rnd; cout << "MS RAND:" << endl << "========" << endl; for( int x = 0; x < 10; x++ ) cout << ms_rnd.rnd() << endl; cout << endl << "BSD RAND:" << endl << "=========" << endl; for( int x = 0; x < 10; x++ ) cout << bsd_rnd.rnd() << endl; cout << endl << endl; system( "pause" ); return 0; }
Output
MS RAND:
========
38
7719
21238
2437
8855
11797
8365
32285
10450
30612
BSD RAND:
=========
12345
1406932606
654583775
1449466924
229283573
1109335178
1051550459
1293799192
794471793
551188310
#! /bin/bash function BSD() { SEED=$(((1103515245 * $SEED + 12345) % 2**31)) echo " $SEED" } function MS() { SEED=$(((214013 * $SEED + 2531011) % 2**31)) echo " $(($SEED / 2**16))" } function output() { SEED=0 echo "$1" for i in {1..10}; do eval "$1" done echo "" }
BSD
12345
1406932606
654583775
1449466924
229283573
1109335178
1051550459
1293799192
794471793
551188310
MS
38
7719
21238
2437
8855
11797
8365
32285
10450
30612