Category Archives: python/pygame
Udemy - Programming Languages
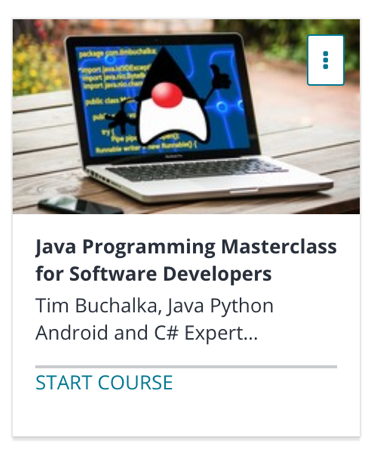
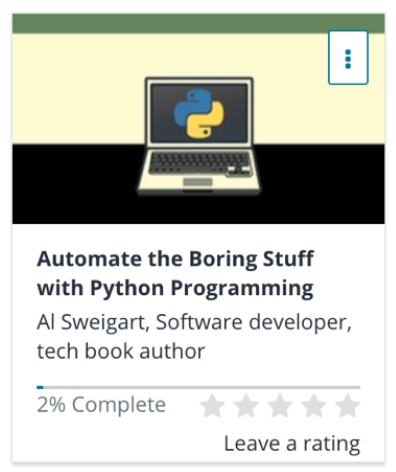
COS 126 Python Language
Python Programs in the Textbook
Booksite Modules
Below is a table of the booksite modules that we use throughout the textbook and booksite and beyond.
SECTION | MODULE | DESCRIPTION |
---|---|---|
1.5 | stdio.py | functions to read/write numbers and text from/to stdin and stdout |
1.5 | stddraw.py | functions to draw geometric shapes |
1.5 | stdaudio.py | functions to create, play, and manipulate sound |
2.2 | stdrandom.py | functions related to random numbers |
2.2 | stdarray.py | functions to create, read, and write 1D and 2D arrays |
2.2 | stdstats.py | functions to compute and plot statistics |
3.1 | color.py | data type for colors |
3.1 | picture.py | data type to process digital images |
3.1 | instream.py | data type to read numbers and text from files and URLs |
3.1 | outstream.py | data type to write numbers and text to files |
If you followed the instructions provided in this booksite (for Windows, Mac OS X, or Linux), then the booksite modules are installed on your computer. If you want to see the source code for the booksite modules, then click on the links in the above table, or download and unzip stdlib-python.zip.
Programs and Data Sets in the Textbook
Below is a table of the Python programs and data sets used in the textbook. Click on the program name to access the Python code; click on the data set name to access the data set; read the textbook for a full discussion. You can download all of the programs as introcs-python.zip and the data as introcs-data.zip.
1 | ELEMENTS OF PROGRAMMING | DATA | |
---|---|---|---|
1.1.1 | helloworld.py | Hello, World | – |
1.1.2 | useargument.py | using a command-line argument | – |
1.2.1 | ruler.py | string concatenation example | – |
1.2.2 | intops.py | integer operators | – |
1.2.3 | floatops.py | float operators | – |
1.2.4 | quadratic.py | quadratic formula | – |
1.2.5 | leapyear.py | leap year | – |
1.3.1 | flip.py | flipping a fair coin | – |
1.3.2 | tenhellos.py | your first loop | – |
1.3.3 | powersoftwo.py | computing powers of two | – |
1.3.4 | divisorpattern.py | your first nested loops | – |
1.3.5 | harmonic.py | harmonic numbers | – |
1.3.6 | sqrt.py | Newton's method | – |
1.3.7 | binary.py | converting to binary | – |
1.3.8 | gambler.py | gambler's ruin simulation | – |
1.3.9 | factors.py | factoring integers | – |
1.4.1 | sample.py | sampling without replacement | – |
1.4.2 | couponcollector.py | coupon collector simulation | – |
1.4.3 | primesieve.py | sieve of Eratosthenes | – |
1.4.4 | selfavoid.py | self-avoiding random walks | – |
1.5.1 | randomseq.py | generating a random sequence | – |
1.5.2 | twentyquestions.py | interactive user input | – |
1.5.3 | average.py | averaging a stream of numbers | – |
1.5.4 | rangefilter.py | a simple filter | – |
1.5.5 | plotfilter.py | standard input to draw filter | usa.txt |
1.5.6 | bouncingball.py | bouncing ball | – |
1.5.7 | playthattune.py | digital signal processing | elise.txt ascale.txt stairwaytoheaven.txt entertainer.txt firstcut.txt freebird.txt looney.txt |
1.6.1 | transition.py | computing the transition matrix | small.txt medium.txt |
1.6.2 | randomsurfer.py | simulating a random surfer | – |
1.6.3 | markov.py | mixing a Markov chain | – |
2 | FUNCTIONS | DATA | |
2.1.1 | harmonicf.py | harmonic numbers (revisited) | – |
2.1.2 | gauss.py | Gaussian functions | – |
2.1.3 | coupon.py | coupon collector (revisited) | – |
2.1.4 | playthattunedeluxe.py | play that tune (revisited) | elise.txt ascale.txt stairwaytoheaven.txt entertainer.txt firstcut.txt freebird.txt looney.txt |
2.2.1 | gaussian.py | Gaussian functions module | – |
2.2.2 | gaussiantable.py | sample Gaussian client | – |
2.2.3 | sierpinski.py | Sierpinski triangle | – |
2.2.4 | ifs.py | iterated function systems | sierpinski.txt barnsley.txt coral.txt culcita.txt cyclosorus.txt dragon.txt fishbone.txt floor.txt koch.txt spiral.txt swirl.txt tree.txt zigzag.txt |
2.2.5 | bernoulli.py | Bernoulli trials | – |
2.3.1 | euclid.py | Euclid's algorithm | – |
2.3.2 | towersofhanoi.py | towers of Hanoi | – |
2.3.3 | beckett.py | Gray code | – |
2.3.4 | htree.py | recursive graphics | – |
2.3.5 | brownian.py | Brownian bridge | – |
2.4.1 | percolationv.py | vertical percolation detection | test5.txt test8.txt |
2.4.2 | percolationio.py | percolation support functions | – |
2.4.3 | visualizev.py | vertical percolation visualization client | – |
2.4.4 | estimatev.py | vertical percolation probability estimate | – |
2.4.5 | percolation.py | percolation detection | test5.txt test8.txt |
2.4.6 | visualize.py | percolation visualization client | – |
2.4.7 | estimate.py | percolation probability estimate | – |
3 | OBJECT ORIENTED PROGRAMMING | DATA | |
3.1.1 | potentialgene.py | potential gene identification | – |
3.1.2 | chargeclient.py | charged particle client | – |
3.1.3 | alberssquares.py | Albers squares | – |
3.1.4 | luminance.py | luminance library | – |
3.1.5 | grayscale.py | converting color to grayscale | mandrill.jpg mandrill.png darwin.jpg darwin.png |
3.1.6 | scale.py | image scaling | mandrill.jpg mandrill.png darwin.jpg darwin.png |
3.1.7 | fade.py | fade effect | mandrill.jpg mandrill.png darwin.jpg darwin.png |
3.1.8 | potential.py | visualizing electric potential | charges.txt |
3.1.9 | cat.py | concatenating files | in1.txt in2.txt |
3.1.10 | stockquote.py | screen scraping for stock quotes | – |
3.1.11 | split.py | splitting a file | djia.csv |
3.2.1 | charge.py | charged-particle data type | – |
3.2.2 | stopwatch.py | stopwatch data type | – |
3.2.3 | histogram.py | histogram data type | – |
3.2.4 | turtle.py | turtle graphics data type | – |
3.2.5 | koch.py | Koch curve | – |
3.2.6 | spiral.py | spira mirabilis | – |
3.2.7 | drunk.py | drunken turtle | – |
3.2.8 | drunks.py | drunken turtles | – |
3.2.9 | complex.py | complex number data type | – |
3.2.10 | mandelbrot.py | Mandelbrot set | – |
3.2.11 | stockaccount.py | stock account data type | turing.txt |
3.3.1 | complexpolar.py | complex numbers (revisited) | – |
3.3.2 | counter.py | counter data type | – |
3.3.3 | vector.py | spatial vector data type | – |
3.3.4 | sketch.py | sketch data type | genome20.txt |
3.3.5 | comparedocuments.py | similarity detection | documents.txt constitution.txt tomsawyer.txt huckfinn.txt prejudice.txt djia.csv amazon.html actg.txt |
3.4.1 | body.py | gravitational body data type | – |
3.4.2 | universe.py | n-body simulation | 2body.txt 3body.txt 4body.txt 2bodytiny.txt |
4 | DATA STRUCTURES | DATA | |
4.1.1 | threesum.py | 3-sum problem | 8ints.txt 1kints.txt 2kints.txt 4kints.txt 8kints.txt 16kints.txt 32kints.txt 64kints.txt 128kints.txt |
4.1.2 | doublingtest.py | validating a doubling hypothesis | – |
4.1.3 | timeops.py | timing operators and functions | – |
4.1.4 | bigarray.py | discovering memory capacity | – |
4.2.1 | questions.py | binary search (20 questions) | – |
4.2.2 | bisection.py | binary search (inverting a function) | – |
4.2.3 | binarysearch.py | binary search (sorted array) | emails.txt white.txt |
4.2.4 | insertion.py | insertion sort | tiny.txt tomsawyer.txt |
4.2.5 | timesort.py | doubling test for sorting functions | – |
4.2.6 | merge.py | mergesort | tiny.txt tomsawyer.txt |
4.2.7 | frequencycount.py | frequency counts | leipzig100k.txt leipzig200k.txt leipzig1m.txt |
4.3.1 | arraystack.py | stack (resizing array implementation) | tobe.txt |
4.3.2 | linkedstack.py | stack (linked list implementation) | tobe.txt |
4.3.3 | evaluate.py | expression evaluation | expression1.txt expression2.txt |
4.3.4 | linkedqueue.py | queue (linked list implementation) | tobe.txt |
4.3.5 | mm1queue.py | M/M/1 queue simulation | – |
4.3.6 | loadbalance.py | load balancing simulation | – |
4.4.1 | lookup.py | dictionary lookup | amino.csv djia.csv elements.csv ip.csv ip-by-country.csv morse.csv phone-na.csv |
4.4.2 | index.py | indexing | mobydick.txt tale.txt |
4.4.3 | hashst.py | hash symbol table data type | – |
4.4.4 | bst.py | BST symbol table data type | – |
4.5.1 | graph.py | graph data type | tinygraph.txt |
4.5.2 | invert.py | using a graph to invert an index | tinygraph.txt movies.txt |
4.5.3 | separation.py | shortest-paths client | routes.txt movies.txt |
4.5.4 | pathfinder.py | shortest-paths client | – |
4.5.5 | smallworld.py | small-world test | tinygraph.txt |
4.5.6 | performer.py | performer-performer graph | tinymovies.txt moviesg.txt |
Computing with SciPy
Transform Science - Enthought
pygame install notes
Error:
In file included from src/scrap.c:28:
/Library/Frameworks/SDL.framework/Versions/Current/Headers/SDL_syswm.h:58:10: fatal error: 'X11/Xlib.h' file not found
#include
^
1 error generated.
error: command '/usr/bin/clang' failed with exit status 1
Repair:
https://stackoverflow.com/questions/14321038/x11-xlib-h-no-such-file-or-directory-on-mac-os-x-mountain-lion
sudo ln -s /opt/X11/include/X11 /usr/local/include/X11
Notes from previous parts of the same install:
Last login: Thu Jun 1 12:40:56 on ttys000
Zanes-MacBook-Air-2:~ Jasper$ /usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
==> This script will install:
/usr/local/bin/brew
/usr/local/share/doc/homebrew
/usr/local/share/man/man1/brew.1
/usr/local/share/zsh/site-functions/_brew
/usr/local/etc/bash_completion.d/brew
/usr/local/Homebrew
Press RETURN to continue or any other key to abort
==> Searching online for the Command Line Tools
==> /usr/bin/sudo /usr/bin/touch /tmp/.com.apple.dt.CommandLineTools.installondemand.in-progress
Password:
^Z
[1]+ Stopped /usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
Zanes-MacBook-Air-2:~ Jasper$
Zanes-MacBook-Air-2:~ Jasper$ ls
Applications Documents Movies Public test1.py
Battleshipinprogress.py Downloads Music SomeLocalDir
Desktop Library Pictures Untitled.py
Zanes-MacBook-Air-2:~ Jasper$ brew install mercurial
Updating Homebrew...
==> Installing dependencies for mercurial: readline, sqlite, gdbm, openssl, python
==> Installing mercurial dependency: readline
xcrun: error: invalid active developer path (/Library/Developer/CommandLineTools), missing xcrun at: /Library/Developer/CommandLineTools/usr/bin/xcrun
Error: Failure while executing: git config --local --replace-all homebrew.private true
Zanes-MacBook-Air-2:~ Jasper$ /usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/uninstall)"
Warning: This script will remove:
/Library/Caches/Homebrew/
/Users/Jasper/Library/Caches/Homebrew/
/usr/local/Cellar/
/usr/local/Homebrew/
/usr/local/Homebrew/.github/
/usr/local/Homebrew/.gitignore
/usr/local/Homebrew/.travis.yml
/usr/local/Homebrew/.yardopts
/usr/local/Homebrew/CODEOFCONDUCT.md
/usr/local/Homebrew/CONTRIBUTING.md
/usr/local/Homebrew/LICENSE.txt
/usr/local/Homebrew/Library/
/usr/local/Homebrew/Library/Homebrew/test/.bundle/
/usr/local/Homebrew/README.md
/usr/local/Homebrew/bin/brew
/usr/local/Homebrew/completions/
/usr/local/Homebrew/docs/
/usr/local/Homebrew/manpages/
/usr/local/bin/brew -> /usr/local/Homebrew/bin/brew
/usr/local/etc/bash_completion.d/brew -> /usr/local/Homebrew/completions/bash/brew
/usr/local/share/doc/homebrew -> /usr/local/Homebrew/docs
/usr/local/share/man/man1/brew-cask.1 -> /usr/local/Homebrew/manpages/brew-cask.1
/usr/local/share/man/man1/brew.1 -> /usr/local/Homebrew/manpages/brew.1
/usr/local/share/zsh/site-functions/_brew -> /usr/local/Homebrew/completions/zsh/_brew
/usr/local/share/zsh/site-functions/_brew_cask -> /usr/local/Homebrew/completions/zsh/_brew_cask
/usr/local/var/homebrew/locks/update
Are you sure you want to uninstall Homebrew? [y/N] y
==> Removing Homebrew installation...
==> Removing empty directories...
==> /usr/bin/sudo /usr/bin/find /usr/local/Cellar /usr/local/Homebrew /usr/local/Frameworks /usr/local/bin /usr/local/etc /usr/local/include /usr/local/lib /usr/local/opt /usr/local/sbin /usr/local/share /usr/local/var -name .DS_Store -delete
==> /usr/bin/sudo /usr/bin/find /usr/local/Cellar /usr/local/Homebrew /usr/local/Frameworks /usr/local/bin /usr/local/etc /usr/local/include /usr/local/lib /usr/local/opt /usr/local/sbin /usr/local/share /usr/local/var -depth -type d -empty -exec rmdir {} ;
==> Homebrew uninstalled!
The following possible Homebrew files were not deleted:
/usr/local/bin/
/usr/local/etc/
/usr/local/share/
/usr/local/var/
You may wish to remove them yourself.
Zanes-MacBook-Air-2:~ Jasper$ /usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
==> This script will install:
/usr/local/bin/brew
/usr/local/share/doc/homebrew
/usr/local/share/man/man1/brew.1
/usr/local/share/zsh/site-functions/_brew
/usr/local/etc/bash_completion.d/brew
/usr/local/Homebrew
==> The following new directories will be created:
/usr/local/Cellar
/usr/local/Homebrew
/usr/local/Frameworks
/usr/local/include
/usr/local/lib
/usr/local/opt
/usr/local/sbin
/usr/local/share/zsh
/usr/local/share/zsh/site-functions
Press RETURN to continue or any other key to abort
==> /usr/bin/sudo /bin/mkdir -p /usr/local/Cellar /usr/local/Homebrew /usr/local/Frameworks /usr/local/include /usr/local/lib /usr/local/opt /usr/local/sbin /usr/local/share/zsh /usr/local/share/zsh/site-functions
Password:
==> /usr/bin/sudo /bin/chmod g+rwx /usr/local/Cellar /usr/local/Homebrew /usr/local/Frameworks /usr/local/include /usr/local/lib /usr/local/opt /usr/local/sbin /usr/local/share/zsh /usr/local/share/zsh/site-functions
==> /usr/bin/sudo /bin/chmod 755 /usr/local/share/zsh /usr/local/share/zsh/site-functions
==> /usr/bin/sudo /usr/sbin/chown Jasper /usr/local/Cellar /usr/local/Homebrew /usr/local/Frameworks /usr/local/include /usr/local/lib /usr/local/opt /usr/local/sbin /usr/local/share/zsh /usr/local/share/zsh/site-functions
==> /usr/bin/sudo /usr/bin/chgrp admin /usr/local/Cellar /usr/local/Homebrew /usr/local/Frameworks /usr/local/include /usr/local/lib /usr/local/opt /usr/local/sbin /usr/local/share/zsh /usr/local/share/zsh/site-functions
==> /usr/bin/sudo /bin/mkdir -p /Users/Jasper/Library/Caches/Homebrew
==> /usr/bin/sudo /bin/chmod g+rwx /Users/Jasper/Library/Caches/Homebrew
==> /usr/bin/sudo /usr/sbin/chown Jasper /Users/Jasper/Library/Caches/Homebrew
==> /usr/bin/sudo /bin/mkdir -p /Library/Caches/Homebrew
==> /usr/bin/sudo /bin/chmod g+rwx /Library/Caches/Homebrew
==> /usr/bin/sudo /usr/sbin/chown Jasper /Library/Caches/Homebrew
==> Searching online for the Command Line Tools
==> /usr/bin/sudo /usr/bin/touch /tmp/.com.apple.dt.CommandLineTools.installondemand.in-progress
^[^[^[Didn't get a response from the Apple Software Update server.
==> Installing
==> /usr/bin/sudo /usr/sbin/softwareupdate -i
Software Update Tool
: No such update
No updates are available.
==> /usr/bin/sudo /bin/rm -f /tmp/.com.apple.dt.CommandLineTools.installondemand.in-progress
==> /usr/bin/sudo /usr/bin/xcode-select --switch /Library/Developer/CommandLineTools
xcode-select: error: invalid developer directory '/Library/Developer/CommandLineTools'
Failed during: /usr/bin/sudo /usr/bin/xcode-select --switch /Library/Developer/CommandLineTools
Zanes-MacBook-Air-2:~ Jasper$ code-select --install
-bash: code-select: command not found
Zanes-MacBook-Air-2:~ Jasper$ xcode-select --install,
xcode-select: error: invalid argument '--install,'
Usage: xcode-select [options]
Print or change the path to the active developer directory. This directory
controls which tools are used for the Xcode command line tools (for example,
xcodebuild) as well as the BSD development commands (such as cc and make).
Options:
-h, --help print this help message and exit
-p, --print-path print the path of the active developer directory
-s
--install open a dialog for installation of the command line developer tools
-v, --version print the xcode-select version
-r, --reset reset to the default command line tools path
Zanes-MacBook-Air-2:~ Jasper$ xcode-select --install
xcode-select: note: install requested for command line developer tools
Zanes-MacBook-Air-2:~ Jasper$ xcode-select --install,
xcode-select: error: invalid argument '--install,'
Usage: xcode-select [options]
Print or change the path to the active developer directory. This directory
controls which tools are used for the Xcode command line tools (for example,
xcodebuild) as well as the BSD development commands (such as cc and make).
Options:
-h, --help print this help message and exit
-p, --print-path print the path of the active developer directory
-s
--install open a dialog for installation of the command line developer tools
-v, --version print the xcode-select version
-r, --reset reset to the default command line tools path
Zanes-MacBook-Air-2:~ Jasper$ brew install mercurial
-bash: /usr/local/bin/brew: No such file or directory
Zanes-MacBook-Air-2:~ Jasper$ ls
Applications Documents Movies Public test1.py
Battleshipinprogress.py Downloads Music SomeLocalDir
Desktop Library Pictures Untitled.py
Zanes-MacBook-Air-2:~ Jasper$ python -version
Unknown option: -e
usage: /Library/Frameworks/Python.framework/Versions/2.7/Resources/Python.app/Contents/MacOS/Python [option] ... [-c cmd | -m mod | file | -] [arg] ...
Try `python -h' for more information.
Zanes-MacBook-Air-2:~ Jasper$ python3 -version
Unknown option: -e
usage: /Library/Frameworks/Python.framework/Versions/3.5/Resources/Python.app/Contents/MacOS/Python [option] ... [-c cmd | -m mod | file | -] [arg] ...
Try `python -h' for more information.
Zanes-MacBook-Air-2:~ Jasper$ brew install mercurial
-bash: /usr/local/bin/brew: No such file or directory
Zanes-MacBook-Air-2:~ Jasper$ hg
-bash: hg: command not found
Zanes-MacBook-Air-2:~ Jasper$ brew install git
-bash: /usr/local/bin/brew: No such file or directory
Zanes-MacBook-Air-2:~ Jasper$ brew info
-bash: /usr/local/bin/brew: No such file or directory
Zanes-MacBook-Air-2:~ Jasper$ show brew
-bash: show: command not found
Zanes-MacBook-Air-2:~ Jasper$ /Developer/usr/bin/xcodebuild -version
-bash: /Developer/usr/bin/xcodebuild: No such file or directory
Zanes-MacBook-Air-2:~ Jasper$ cd /Developer/usr/bin/
-bash: cd: /Developer/usr/bin/: No such file or directory
Zanes-MacBook-Air-2:~ Jasper$ cd ~/Developer/usr/bin/xcodebuild -version
-bash: cd: /Users/Jasper/Developer/usr/bin/xcodebuild: No such file or directory
Zanes-MacBook-Air-2:~ Jasper$ ls
Applications Documents Movies Public test1.py
Battleshipinprogress.py Downloads Music SomeLocalDir
Desktop Library Pictures Untitled.py
Zanes-MacBook-Air-2:~ Jasper$ pwd
/Users/Jasper
Zanes-MacBook-Air-2:~ Jasper$ cd ..
Zanes-MacBook-Air-2:Users Jasper$ ls
Guest Jasper Shared ZaneScott matthewscott
Zanes-MacBook-Air-2:Users Jasper$ cd ..
Zanes-MacBook-Air-2:/ Jasper$ ls
Applications bin private
Developer cores sbin
Library dev tmp
Network etc usr
System home var
User Information installer.failurerequests vm
Users net
Volumes opt
Zanes-MacBook-Air-2:/ Jasper$ cd /Developer/usr/bin/
-bash: cd: /Developer/usr/bin/: No such file or directory
Zanes-MacBook-Air-2:/ Jasper$ cd Developer/
Zanes-MacBook-Air-2:Developer Jasper$ cd usr
-bash: cd: usr: No such file or directory
Zanes-MacBook-Air-2:Developer Jasper$ ls
Python
Zanes-MacBook-Air-2:Developer Jasper$ cd ..
Zanes-MacBook-Air-2:/ Jasper$ ls
Applications bin private
Developer cores sbin
Library dev tmp
Network etc usr
System home var
User Information installer.failurerequests vm
Users net
Volumes opt
Zanes-MacBook-Air-2:/ Jasper$ cd Developer/
Zanes-MacBook-Air-2:Developer Jasper$ ls
Python
Zanes-MacBook-Air-2:Developer Jasper$ cd ..
Zanes-MacBook-Air-2:/ Jasper$ ls
Applications bin private
Developer cores sbin
Library dev tmp
Network etc usr
System home var
User Information installer.failurerequests vm
Users net
Volumes opt
Zanes-MacBook-Air-2:/ Jasper$ cd bin
Zanes-MacBook-Air-2:bin Jasper$ ls
[ date expr ln pwd sync
bash dd hostname ls rm tcsh
cat df kill mkdir rmdir test
chmod domainname ksh mv sh unlink
cp echo launchctl pax sleep wait4path
csh ed link ps stty zsh
Zanes-MacBook-Air-2:bin Jasper$ xcodebuild -version
xcode-select: error: tool 'xcodebuild' requires Xcode, but active developer directory '/Library/Developer/CommandLineTools' is a command line tools instance
Zanes-MacBook-Air-2:bin Jasper$ xcode-select
xcode-select: error: no command option given
Usage: xcode-select [options]
Print or change the path to the active developer directory. This directory
controls which tools are used for the Xcode command line tools (for example,
xcodebuild) as well as the BSD development commands (such as cc and make).
Options:
-h, --help print this help message and exit
-p, --print-path print the path of the active developer directory
-s
--install open a dialog for installation of the command line developer tools
-v, --version print the xcode-select version
-r, --reset reset to the default command line tools path
Zanes-MacBook-Air-2:bin Jasper$ xcode-select -v
xcode-select version 2347.
Zanes-MacBook-Air-2:bin Jasper$ xcode-build
-bash: xcode-build: command not found
Zanes-MacBook-Air-2:bin Jasper$ xcode-select --install
xcode-select: note: install requested for command line developer tools
Zanes-MacBook-Air-2:bin Jasper$ xcode-select --install
xcode-select: note: install requested for command line developer tools
Zanes-MacBook-Air-2:bin Jasper$ ls
[ date expr ln pwd sync
bash dd hostname ls rm tcsh
cat df kill mkdir rmdir test
chmod domainname ksh mv sh unlink
cp echo launchctl pax sleep wait4path
csh ed link ps stty zsh
Zanes-MacBook-Air-2:bin Jasper$ pwd
/bin
Zanes-MacBook-Air-2:bin Jasper$ /usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
==> This script will install:
Zanes-MacBook-Air-2:bin Jasper$ brew info xlib
Error: No available formula with the name "xlib"
==> Searching for a previously deleted formula...
Error: No previously deleted formula found.
Zanes-MacBook-Air-2:bin Jasper$ brew search xlib
No formula found for "xlib".
Zanes-MacBook-Air-2:bin Jasper$ brew search xlib
^C
Zanes-MacBook-Air-2:bin Jasper$ pip3 install pygame
IDLE warns against an old TCL version
$ echo $PATH /Library/Frameworks/Python.framework/Versions/3.3/bin:/usr/bin:/bin:/usr/sbin:/sbin:/usr/local/bin $ which tclsh /usr/bin/tclsh $ ls -l /usr/bin/tclsh* lrwxr-xr-x 1 root wheel 17 Jan 15 10:45 /usr/bin/tclsh -> /usr/bin/tclsh8.6 lrwxr-xr-x 1 root wheel 67 Oct 25 19:59 /usr/bin/tclsh8.4 -> ../../System/Library/Frameworks/Tcl.framework/Versions/8.4/tclsh8.4 lrwxr-xr-x 1 root wheel 67 Oct 25 19:59 /usr/bin/tclsh8.5 -> ../../System/Library/Frameworks/Tcl.framework/Versions/8.5/tclsh8.5 lrwxr-xr-x 1 root wheel 23 Jan 15 10:45 /usr/bin/tclsh8.6 -> /usr/local/bin/tclsh8.6 $ ls -l /usr/local/bin/tclsh* lrwxr-xr-x 1 root admin 8 Jan 15 10:40 /usr/local/bin/tclsh -> tclsh8.6 -rwxr-xr-x 1 root admin 41716 Oct 27 04:45 /usr/local/bin/tclsh8.6
According to the "How Python Chooses Which Tk Library To Use" section of the "IDLE and tinter with Tcl/Tk on Mac OS X" page on the official python website,
The Python for Mac OS X installers downloaded from this website dynamically link at runtime to Tcl/Tk Mac OS X frameworks. The Tcl/Tk major version is determined when the installer is created and cannot be overridden. The Python 64-bit/32-bit Mac OS X installers for Python 3.4.x, 3.3.x, 3.2.x, and 2.7.x dynamically link to Tcl/Tk 8.5 frameworks.
So it seems that
The current python installations for OS X do not recognize the latest ActiveTcl version (namely 8.6).
The Tcl/Tk version used by python is hard-wired during the python installation procedure and cannot be changed later.
From these observation the solution is clear:
Install the latest 8.5 ActiveTcl version.
Reinstall python.
I have followed these steps and now everything seems to work.
Deitel Python Resource Center Tutorials
Make Yahoo! Web Service REST calls with Python
Deitel - Game Programming resources
Python Programming Professional Bundle
Java and python assessments
Python Programming Professional Bundle
Python Programming Professional Bundle
Mastering Object-oriented Python
Practical Maya programming with Python
16 Common Python Runtime Errors Beginners Find
Program Arcade Games With Python And Pygame
How to Think Like a Computer Scientist: Learning with Python 3
python/pygame mancala game
Python Programs Intermediate
Python Tutorials for beginners
Beginning Python Tutorials from a 13-year-old
Python tutorials 21-30 The New Boston Forum
An Introduction to Interactive Programming in Python (Part 1)
pygame issues
Skulpt
bitbucket - pygame
2048 game at coursera
Codesculptor
pygame installation in PC
https://pypi.python.org/pypi/pip
select "pip-7.0.3.tar.gz (md5, pgp)" and un-compress it.
c:\Users\Jane\Downloads\pip-7.0.3\pip-7.0.3>c:\Python34\python.exe setup.py install
This is inside the folder pip-7.0.3>pip-7.0.3 where there is a file setup.py
Start here is pip is already installed:
from pygame.org/download.shtml click on the bottom link before the unix distribution
Choose last file:
pygame-1.9.2a0-cp34-none-win32.whl
c:\python34>Scripts\pip.exe install (drag from wherever the file is)pygame-1.9.2a0-cp34-none-win32.whl
fizzbuzz in Python using random, how does it work?
How to think like a computer scientist
Mathematics for the digital age
pygame-rect collision response
trinket on the web
interactive python on the web
Battleship in GitHub
Python 3 Formatted output
learn python
informal intro to pyhton 3
CSTA standards - September 2014
CSTA workshops
Bits of Binary
trinket - python
pygame installation for mac
Published on August 28, 2016
Published on May 7, 2014
From youtube.com: How to install Python3 + Pygame on mac os x - Mac computer
I hope the video was helpful, drop me a comment if you have any questions or help..
Xcode - https://itunes.apple.com/gb/app/xcode...
http://matthewcarriere.com/2013/08/05/how-to-install-and-use-homebrew/
Python3 - https://www.python.org/
tcltk versions - http://www.activestate.com/activetcl/...
DOCUMENT!!!
Install Python 3
Homebrew has a recipe for Python 3, which we are going to use. Type
brew install python3
and hit Return. Once that is done, verify the Python version by typing
python3 —version
Install Pygame
First install the Mercurial version control system:
brew install mercurial
Note: It didn't work for me at first. To test if it works for you, type:
hg
and press enter. If you don't get an error but a text that starts with Mercurial Distributed SCM, you have no problem. If you do get an error, type again
brew install mercurial
and press enter. Hopefully it will work.
Then do the same for the git version control system, which is needed by a dependency package:
brew install git
Now install all the dependencies of Pygame:
brew install sdl sdl_image sdl_mixer sdl_ttf portmidi
And now, finally:
pip3 install hg+http://bitbucket.org/pygame/pygame
After this is done, verify that it is working:
python3
At the prompt, type:
import pygame
More...